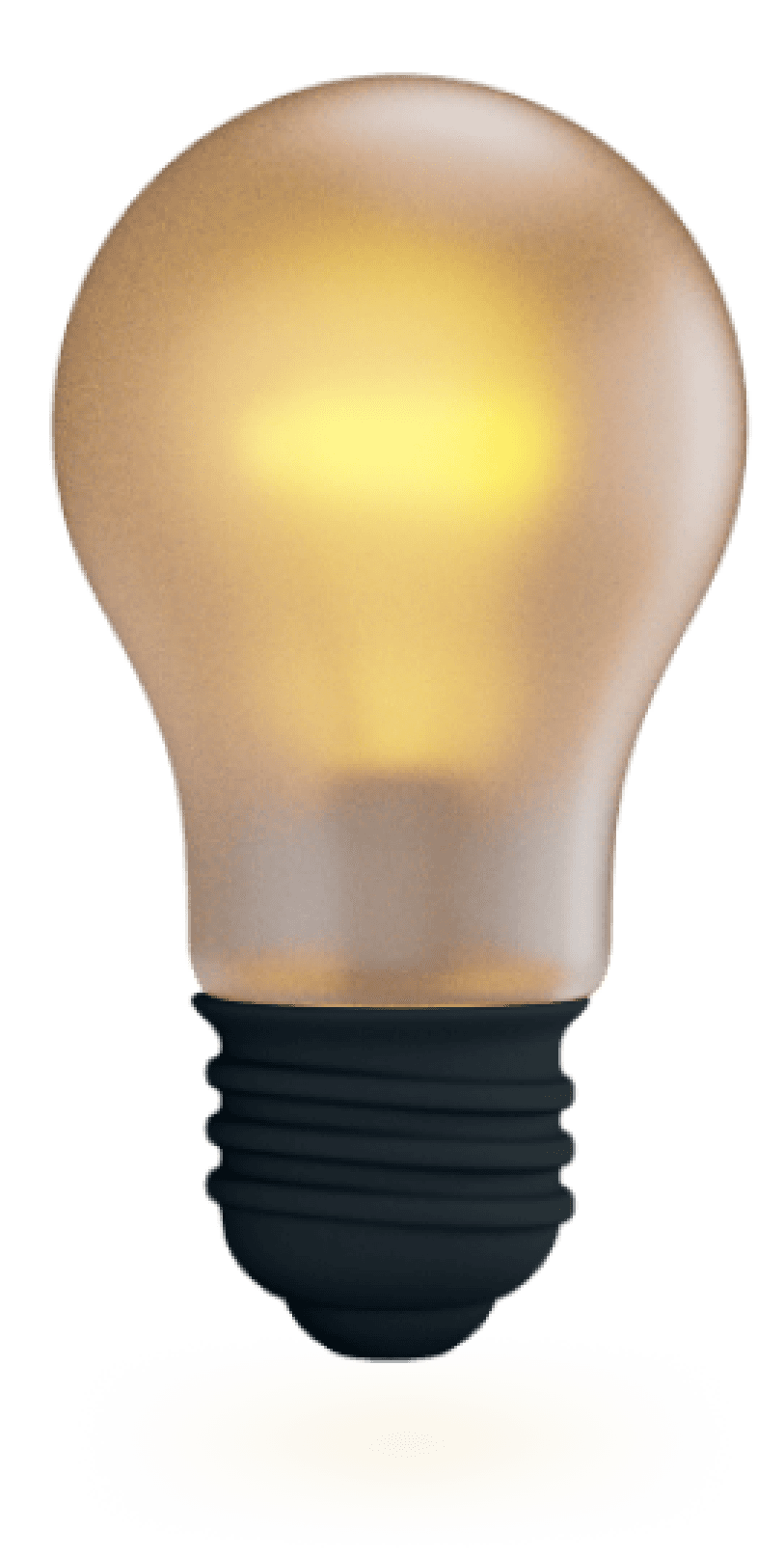
Infrastructure
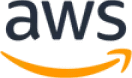




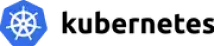
Frontend
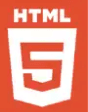
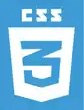
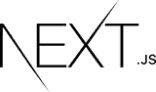
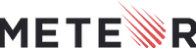
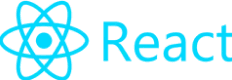
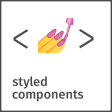
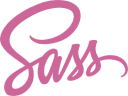
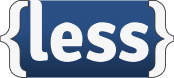
Backend
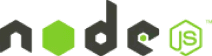
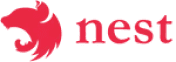
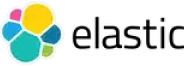
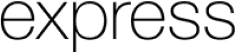
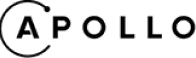
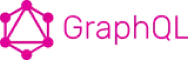
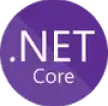
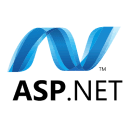
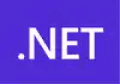
Databases
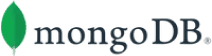
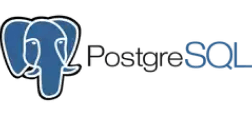
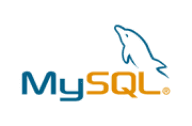
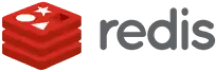
Testing
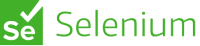
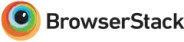
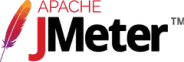

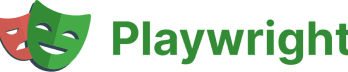
Mobile
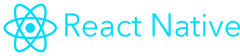
Programming languages


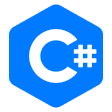
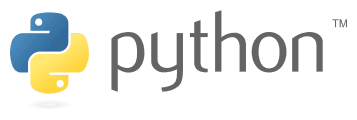
AI
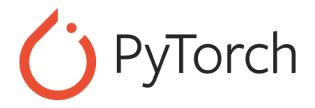
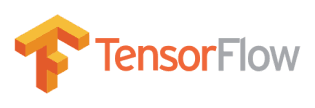
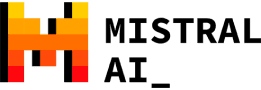
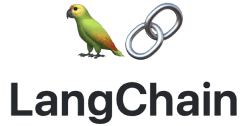
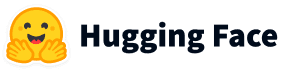
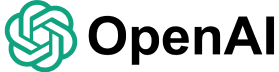
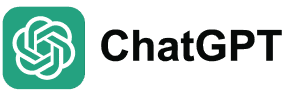
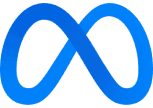
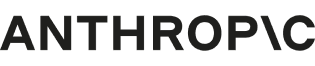
Partners & Clients
It's our honor and pleasure to have contributed to our partners' and clients' success
It's our honor and pleasure to have contributed to our partners' and clients' success